Minting
Immutable's SDK simplifies token minting for content creators, allowing them to mint tokens from their deployed NFT collections.
This guide covers the core concepts of minting and provides references to guides on how to effectively mint on Immutable zkEVM.
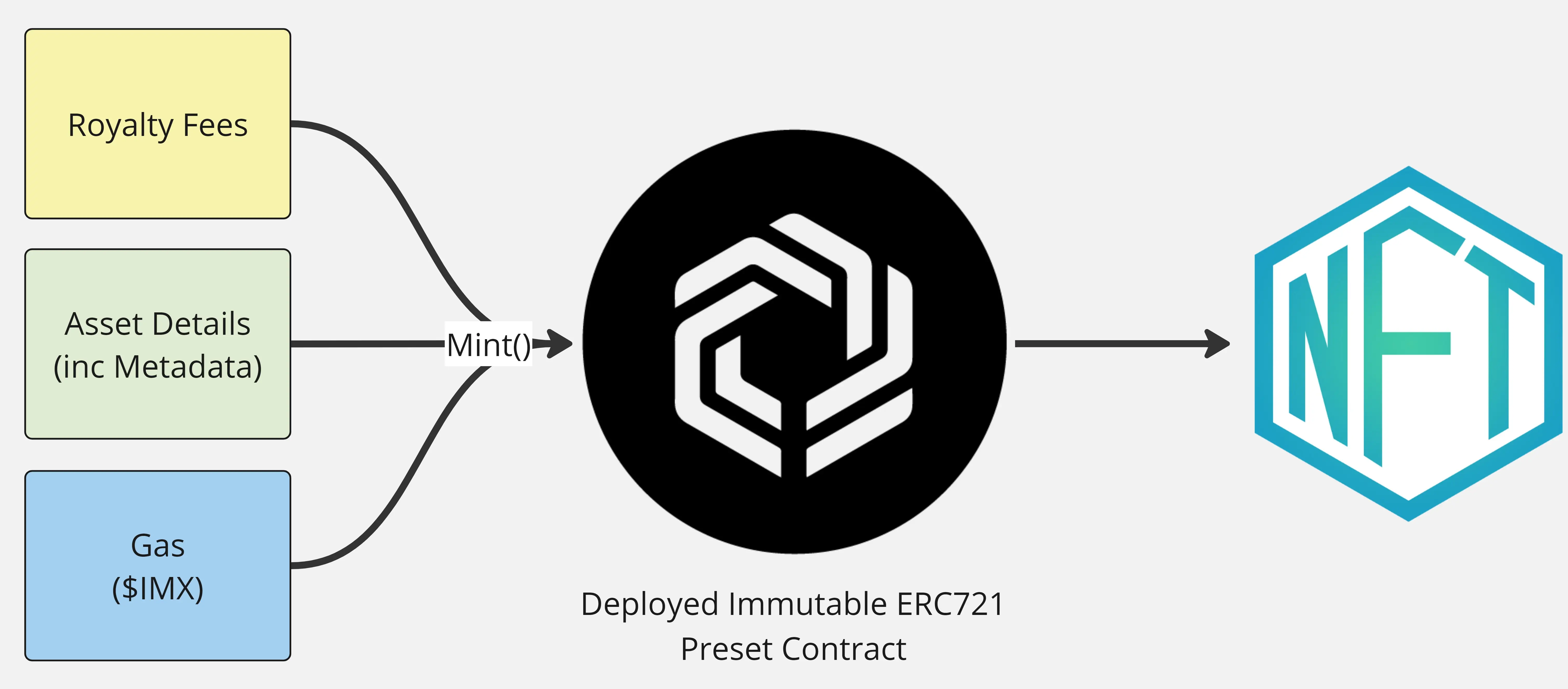
For a step-by-step guide on how to mint an NFT on zkEVM, including contract deployment, check out our Create game assets tutorial.
What is minting?
Minting an NFT (non-fungible token) involves creating a unique digital asset on the blockchain, representing items like art, music, videos, or collectibles, whose ownership and authenticity can be verified.
In Web3 gaming, minting often refers to generating and assigning in-game assets to players.
Why do I need to deploy a smart contract before I can mint tokens?
Before minting an NFT (non-fungible token), the first step is to deploy an ERC721 contract on the blockchain. Smart contracts serve as essential blueprints for the NFTs they create, encompassing a range of functions that facilitate the maintenance of the collection and its associated tokens. Among these functions is the capability to mint new NFTs.
ERC721 contracts are designed specifically for managing NFTs, offering a set of rules and functions that govern the creation, ownership, and transfer of unique digital assets. These contracts play a crucial role in enabling the seamless handling of non-fungible tokens, ensuring their uniqueness, scarcity, and ownership rights within the blockchain ecosystem.
More information:
- ERC721 token standard
- Immutable's contract presets (containing the template ERC721 contract that can be deployed)
Minting and gas fees
Deploying new ERC721 contracts and minting NFTs from these contracts update the blockchain, resulting in gas fees denominated in a unit called "gas." Gas represents the computational effort needed to process and validate transactions, execute smart contracts, and perform other operations on the network.
Gas fees serve several vital purposes:
- Preventing network abuse: By imposing a cost on executing operations, gas fees discourage malicious actors from overloading the network with excessive or spam transactions.
- Resource allocation: Gas fees ensure that resources, such as computational power and storage, are appropriately allocated to process transactions and execute smart contracts, maintaining network efficiency.
- Incentivizing miners/validators: Miners or validators who include transactions in a block and validate them receive gas fees as a reward for their work. This incentivizes participants to validate transactions, supporting network security and integrity.
To mint a new NFT, a wallet is required to cover the associated gas fees. It's worth noting that the minting method you choose can influence the amount of gas required for various actions.
How do I mint an NFT from a deployed ERC721 contract?
Check out the Mint tokens step in our Create game assets tutorial for full instructions on how to mint tokens from a deployed smart contract.
How do I batch mint an NFT from a deployed ERC721 contract?
Minting in batches if more efficent than single mint requests and can end up saving a game studio and player significant gas fees.
Immutable's contract presets contains the mintBatch([CONTRACT_ADDRESS, TOKEN_ID])
which accepts an array of wallet addresses and Token IDs to mint a batch of NFTs with a single request.
To use the batch mint function, deploy Immutable's contract presets; then create the following script
CONTRACT_ADDRESS
- The address of the deployed preset contractPRIVATE_KEY
- The private key of the wallet that is paying the gas fees of the mint request
- Typescript
import { getDefaultProvider, Wallet } from 'ethers';
import { Provider, TransactionResponse } from '@ethersproject/providers';
import { ERC721Client } from '@imtbl/contracts';
const CONTRACT_ADDRESS = 'CONTRACT_ADDRESS';
const PRIVATE_KEY = 'PRIVATE_KEY';
const provider = getDefaultProvider('https://rpc.testnet.immutable.com');
const ANOTHER_RECIPIENT = 'RECIPIENT_ADDRESS';
const batchMint = async (provider: Provider): Promise<TransactionResponse> => {
// Bound contract instance
const contract = new ERC721Client(CONTRACT_ADDRESS);
// The wallet of the intended signer of the mint request
const wallet = new Wallet(PRIVATE_KEY, provider);
// We can use the read function hasRole to check if the intended signer
// has sufficient permissions to mint before we send the transaction
const minterRole = await contract.MINTER_ROLE(provider);
const hasMinterRole = await contract.hasRole(
provider,
minterRole,
wallet.address
);
if (!hasMinterRole) {
// Handle scenario without permissions...
console.log('Account doesnt have permissions to mint.');
return Promise.reject(
new Error('Account doesnt have permissions to mint.')
);
}
// Specify who we want to receive the minted token
const recipient = wallet.address;
// Construct list of tokens to mint
const mintRequests = [
{
to: recipient,
tokenIds: [2, 3, 4],
},
{
to: ANOTHER_RECIPIENT,
tokenIds: [5, 6, 7],
},
];
// Rather than be executed directly, contract write functions on the SDK client are returned
// as populated transactions so that users can implement their own transaction signing logic.
const populatedTransaction = await contract.populateSafeMintBatch(
mintRequests
);
const result = await wallet.sendTransaction(populatedTransaction);
console.log(result); // To get the TransactionResponse value
return result;
};
batchMint(provider);
Congratulations you have just created your first batch of NFTs with Token ID 2, 3 & 4 to the CONTRACT_ADDRESS
wallet specified.
For more information on batch minting, have a look at our Batch Minting product guide
What happens once an NFT is minted?
Once an NFT is successfully minted, it becomes a permanent record on the blockchain. While on-chain data is publicly accessible, off-chain metadata remains private. Upon successful minting, Immutable's search engine is activated, indexing the NFT's metadata for efficient retrieval and discovery.
For additional details on Immutable's indexer, please refer to our Blockchain Data guide.